如何高效地使用API来读取位图数据?
读取位图数据通常涉及使用图像处理库或API来加载和解析位图文件,以下是一个详细的步骤,介绍如何使用Python中的Pillow库(PIL)来读取位图数据:
安装Pillow库
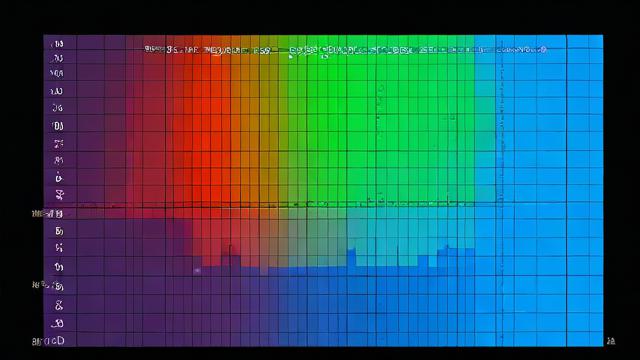
确保你已经安装了Pillow库,如果没有安装,可以使用以下命令进行安装:
pip install Pillow
导入必要的模块
在你的Python脚本中导入Pillow库:
from PIL import Image import numpy as np
读取位图文件
使用Pillow库打开并读取位图文件:
打开位图文件 image = Image.open('path_to_your_bitmap_file.bmp')
获取图像信息
你可以获取图像的一些基本信息,例如尺寸和模式:
print("Image size:", image.size) # 输出图像尺寸 (宽度, 高度) print("Image mode:", image.mode) # 输出图像模式 ('1' for 1-bit pixels, 'L' for 8-bit pixels, etc.)
将图像转换为NumPy数组
为了更方便地处理图像数据,可以将图像转换为NumPy数组:
将图像转换为NumPy数组 image_array = np.array(image) print("Image array shape:", image_array.shape)
访问和操作像素数据
你可以访问和操作图像的像素数据,获取某个像素的值:
获取某个像素的值 (假设图像是灰度图像) x, y = 10, 20 pixel_value = image_array[y, x] print("Pixel value at ({}, {}):".format(x, y), pixel_value)
修改像素值并保存图像
你也可以修改像素值并将修改后的图像保存回来:
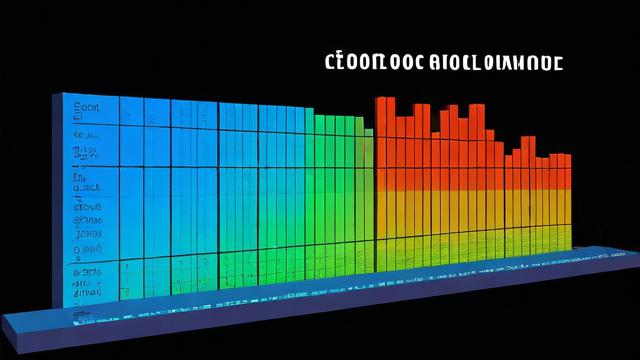
修改某个像素的值 image_array[y, x] = 255 # 将该像素设置为白色 创建一个新的图像对象 modified_image = Image.fromarray(image_array) 保存修改后的图像 modified_image.save('modified_bitmap_file.bmp')
完整示例代码
以下是一个完整的示例代码,演示如何读取、处理和保存位图数据:
from PIL import Image import numpy as np 打开位图文件 image = Image.open('path_to_your_bitmap_file.bmp') 获取图像信息 print("Image size:", image.size) print("Image mode:", image.mode) 将图像转换为NumPy数组 image_array = np.array(image) print("Image array shape:", image_array.shape) 获取某个像素的值 (假设图像是灰度图像) x, y = 10, 20 pixel_value = image_array[y, x] print("Pixel value at ({}, {}):".format(x, y), pixel_value) 修改某个像素的值 image_array[y, x] = 255 # 将该像素设置为白色 创建一个新的图像对象 modified_image = Image.fromarray(image_array) 保存修改后的图像 modified_image.save('modified_bitmap_file.bmp')
通过以上步骤,你可以成功地读取、处理和保存位图数据,如果你需要处理不同类型的位图(例如彩色图像),可以根据具体需求调整代码。
以上就是关于“api读取位图数据”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
-- 展开阅读全文 --
新手求教:如何找到我的亚马逊账号ID?求高人指点,急等!