如何在ArcGIS JS中实现点的标注功能?
ArcGIS JS中的点标注
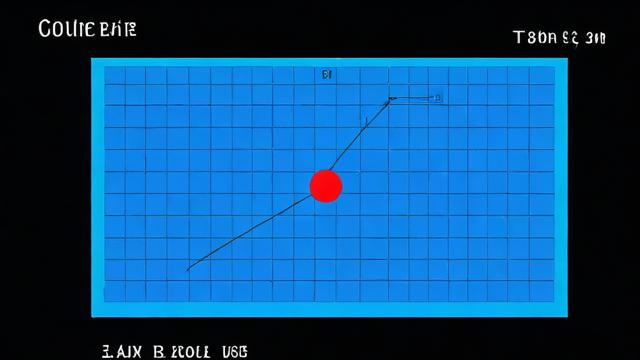
背景介绍
ArcGIS JS API是Esri公司推出的一个强大的开发工具包,用于在Web应用中实现地理信息系统(GIS)的可视化和分析功能,通过使用ArcGIS JS API,开发者可以轻松地将地图、图层、标注等功能集成到他们的Web应用中,本文将详细介绍如何在ArcGIS JS中实现点的标注,包括数据准备、后台接口设计、前端交互以及相关代码示例。
基本概念
点标注:指在地图上对特定位置的点进行标识和说明,通常用于标记兴趣点、事件地点等。
GraphicsLayer:用于绘制图形元素(如点、线、面)的图层。
Symbol:符号类,用于定义点标注的样式,包括颜色、形状、大小等属性。
PopupTemplate:弹出窗口模板,用于定义点击标注点时显示的信息内容和格式。
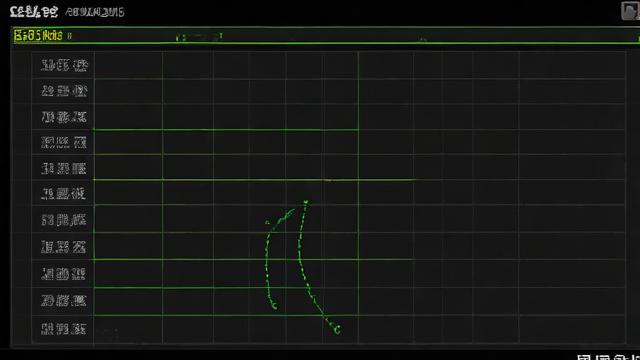
数据准备
数据库表结构
在进行点标注之前,首先需要准备好存储点信息的数据表,以下是一个示例表结构:
字段名 | 描述 |
id | 主键 |
pointName | 名称 |
description | 描述 |
longitude | 经度 |
latitude | 纬度 |
spatialReference | 空间坐标系 |
createTime | 创建时间 |
示例数据
假设我们有以下几条示例数据:
[ { "id": 1, "pointName": "Location1", "description": "This is the first location", "longitude": -122.68, "latitude": 37.81, "spatialReference": { "wkid": 4326 }, "createTime": "2024-07-17T12:00:00Z" }, { "id": 2, "pointName": "Location2", "description": "This is the second location", "longitude": -122.69, "latitude": 37.82, "spatialReference": { "wkid": 4326 }, "createTime": "2024-07-18T12:00:00Z" } ]
后台接口设计
后台接口主要用于增删改查点数据,以下是一个简单的示例:
添加点:POST /addPoint
删除点:DELETE /deletePoint/{id}
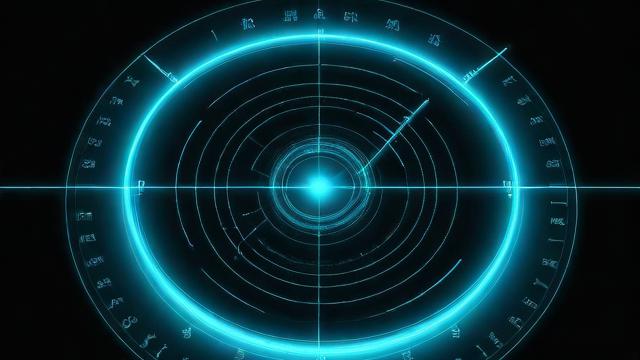
修改点:PUT /updatePoint/{id}
查询所有点:GET /getAllPoints
前端实现
引入必要的库
确保你已经在项目中引入了ArcGIS JS API的相关库。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>ArcGIS JS Point Labeling</title> <link rel="stylesheet" href="https://js.arcgis.com/4.25/esri/themes/light/main.css"> <script src="https://js.arcgis.com/4.25/"></script> <style> #viewDiv { padding: 0; margin: 0; height: 100vh; } </style> </head> <body> <div id="viewDiv"></div> <script> require([ "esri/Map", "esri/views/MapView", "esri/Graphic", "esri/layers/GraphicsLayer", "esri/symbols/SimpleMarkerSymbol", "esri/symbols/TextSymbol", "esri/PopupTemplate", "esri/widgets/Popup" ], function(Map, MapView, Graphic, GraphicsLayer, SimpleMarkerSymbol, TextSymbol, PopupTemplate, Popup) { const map = new Map({ basemap: "streets" }); const view = new MapView({ container: "viewDiv", map: map, center: [-122.68, 37.81], // Longitude, Latitude zoom: 12 }); const graphicsLayer = new GraphicsLayer(); map.add(graphicsLayer); const simpleMarkerSymbol = new SimpleMarkerSymbol(); const textSymbol = new TextSymbol({ text: "Label", color: "black", haloColor: "white", haloSize: "1px", font: { size: 12, weight: "bold", family: "sans-serif" } }); const popupTemplate = new PopupTemplate({ title: "My Point", content: "This is a labeled point" }); function addPointToMap(longitude, latitude, name, description) { const point = { type: "point", longitude: longitude, latitude: latitude, spatialReference: { wkid: 4326 } }; const graphic = new Graphic({ geometry: point, symbol: simpleMarkerSymbol, attributes: { name: name, description: description, popupTemplate: popupTemplate }, popupTemplate: popupTemplate }); graphicsLayer.add(graphic); } // 示例:添加两个点 addPointToMap(-122.68, 37.81, "Location1", "This is the first location"); addPointToMap(-122.69, 37.82, "Location2", "This is the second location"); }); </script> </body> </html>
上述代码实现了一个简单的地图应用,其中包含两个标注点,用户可以通过修改addPointToMap
函数来添加更多的点。
动态添加和编辑点标注
为了实现动态添加和编辑点标注,可以使用ArcGIS JS API提供的Draw
小部件,以下是一个完整的示例:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>ArcGIS JS Point Labeling with Interaction</title> <link rel="stylesheet" href="https://js.arcgis.com/4.25/esri/themes/light/main.css"> <script src="https://js.arcgis.com/4.25/"></script> <style> #viewDiv { padding: 0; margin: 0; height: 100vh; } </style> </head> <body> <div id="viewDiv"></div> <script> require([ "esri/Map", "esri/views/MapView", "esri/Graphic", "esri/layers/GraphicsLayer", "esri/symbols/SimpleMarkerSymbol", "esri/symbols/TextSymbol", "esri/PopupTemplate", "esri/widgets/Draw", "esri/widgets/Popup" ], function(Map, MapView, Graphic, GraphicsLayer, SimpleMarkerSymbol, TextSymbol, PopupTemplate, Draw, Popup) { const map = new Map({ basemap: "streets" }); const view = new MapView({ container: "viewDiv", map: map, center: [-122.68, 37.81], // Longitude, Latitude zoom: 12 }); const graphicsLayer = new GraphicsLayer(); map.add(graphicsLayer); const simpleMarkerSymbol = new SimpleMarkerSymbol(); const textSymbol = new TextSymbol({ text: "Label", color: "black", haloColor: "white", haloSize: "1px", font: { size: 12, weight: "bold", family: "sans-serif" } }); const popupTemplate = new PopupTemplate({ title: "{{name}}", content: "{{description}}" }); const draw = new Draw({ view: view, layer: graphicsLayer, drawTooltips: true // 显示绘制工具提示 }); view.ui.empty("top-left").add(draw.toolbar); // 将绘制工具添加到视图顶部左侧的位置 view.ui.empty("top-right").add(new Popup({ view: view })); // 添加弹出窗口小部件 draw.on("draw-end", function(event) { const geoStr = JSON.stringify(event.geometry); // 获取绘制结束的几何对象并转换为字符串 const name = document.getElementById("pointName").value; // 获取名称输入框的值 const description = document.getElementById("pointDecrib").value; // 获取描述输入框的值 if (name === "" || description === "") { alert("不允许输入空值!"); // 如果名称或描述为空,则弹出警告框 return; } // 发送Ajax请求保存点数据到数据库中(这里省略具体实现) // $.get("markerPoint/addPoint", {...}, function(result){ ... }); // 清空临时图形图层并重新绘制图形(模拟保存成功) graphicsLayer.removeAll(); // 清空临时图形图层 const point = JSON.parse(geoStr); // 解析几何对象字符串为JSON对象 const graphic = new Graphic({ geometry: point, symbol: simpleMarkerSymbol, attributes: { name: name, description: description, popupTemplate: popupTemplate }, popupTemplate: popupTemplate }); graphicsLayer.add(graphic); // 将新图形添加到图形图层中 }); }); </script> </body> </html>
各位小伙伴们,我刚刚为大家分享了有关“arcgis js 点 标注”的知识,希望对你们有所帮助。如果您还有其他相关问题需要解决,欢迎随时提出哦!
暂无评论,2人围观