如何在JSP中实现分页查询功能?
分页查询JSP代码实现
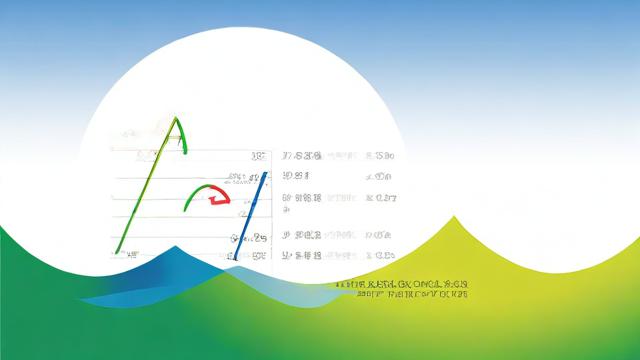
在Web开发中,分页查询是一种常见的需求,通过分页查询,可以将大量数据分割成多个页面进行展示,提高用户体验和系统性能,本文将介绍如何在JSP(Java Server Pages)中实现分页查询功能,包括数据库设计、后端逻辑以及前端展示。
一、数据库设计
我们需要一个包含数据的数据库表,假设我们有一个简单的用户表users
,结构如下:
id | name | |
1 | Alice | alice@example.com |
2 | Bob | bob@example.com |
... | ... | ... |
创建数据库和表
CREATE DATABASE PaginationDemo; USE PaginationDemo; CREATE TABLE users ( id INT PRIMARY KEY AUTO_INCREMENT, name VARCHAR(50), email VARCHAR(100) );
二、后端逻辑(Servlet)
我们需要编写一个Servlet来处理分页查询请求,这个Servlet将接收当前页码和每页显示的记录数作为参数,并从数据库中检索相应的数据。
分页查询Servlet代码
import java.io.IOException; import java.sql.*; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; @WebServlet("/paginate") public class PaginateServlet extends HttpServlet { private static final int RECORDS_PER_PAGE = 10; // 每页显示的记录数 protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { int currentPage = Integer.parseInt(request.getParameter("page")); int offset = (currentPage 1) * RECORDS_PER_PAGE; try (Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/PaginationDemo", "root", "password"); Statement stmt = conn.createStatement()) { // 计算总记录数 String countQuery = "SELECT COUNT(*) FROM users"; ResultSet rs = stmt.executeQuery(countQuery); int totalRecords = 0; if (rs.next()) { totalRecords = rs.getInt(1); } rs.close(); // 计算总页数 int totalPages = (int) Math.ceil((double) totalRecords / RECORDS_PER_PAGE); // 获取当前页的数据 String dataQuery = "SELECT * FROM users LIMIT ? OFFSET ?"; PreparedStatement pstmt = conn.prepareStatement(dataQuery); pstmt.setInt(1, RECORDS_PER_PAGE); pstmt.setInt(2, offset); rs = pstmt.executeQuery(); request.setAttribute("users", rs); request.setAttribute("totalPages", totalPages); request.setAttribute("currentPage", currentPage); rs.close(); pstmt.close(); } catch (SQLException e) { e.printStackTrace(); } request.getRequestDispatcher("/paginate.jsp").forward(request, response); } }
三、前端展示(JSP)
我们需要一个JSP页面来展示分页查询的结果,该页面将从请求属性中获取用户列表、总页数和当前页码,并进行相应的展示。
paginate.jsp代码
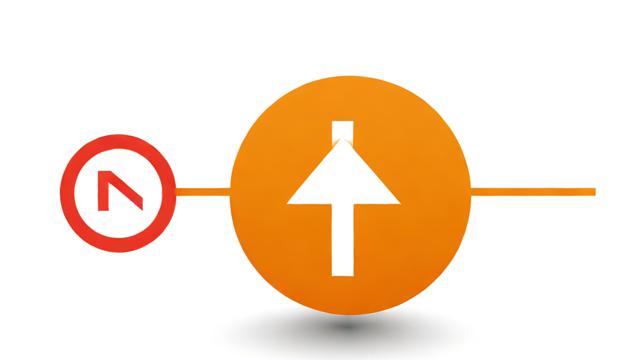
<%@ page contentType="text/html;charset=UTF-8" language="java" %> <!DOCTYPE html> <html> <head> <title>分页查询示例</title> <style> table { width: 100%; border-collapse: collapse; } th, td { border: 1px solid #ddd; padding: 8px; text-align: left; } th { background-color: #f2f2f2; } .pagination { margin-top: 20px; text-align: center; } .pagination a { margin: 0 5px; text-decoration: none; color: blue; } </style> </head> <body> <h1>用户列表</h1> <table> <thead> <tr> <th>ID</th> <th>姓名</th> <th>邮箱</th> </tr> </thead> <tbody> <c:forEach var="user" items="${requestScope.users}"> <tr> <td>${user.id}</td> <td>${user.name}</td> <td>${user.email}</td> </tr> </c:forEach> </tbody> </table> <div class="pagination"> <c:if test="${requestScope.currentPage > 1}"> <a href="paginate?page=${requestScope.currentPage 1}">上一页</a> </c:if> <c:forEach begin="1" end="${requestScope.totalPages}" var="i"> <a href="paginate?page=${i}">${i}</a> </c:forEach> <c:if test="${requestScope.currentPage < requestScope.totalPages}"> <a href="paginate?page=${requestScope.currentPage + 1}">下一页</a> </c:if> </div> </body> </html>
四、相关问题与解答
问题1:如何更改每页显示的记录数?
解答:可以通过修改PaginateServlet
中的RECORDS_PER_PAGE
常量来更改每页显示的记录数,将RECORDS_PER_PAGE
设置为 15,则每页将显示15条记录,前端展示部分无需任何修改即可适应新的记录数。
问题2:如何处理数据库连接失败的情况?
解答:在PaginateServlet
中,如果数据库连接失败(由于数据库服务器不可用或凭据错误),会抛出SQLException
,为了处理这种情况,可以在catch
块中添加适当的错误处理逻辑,例如记录错误日志、向用户显示友好的错误消息等,还可以考虑使用连接池来管理数据库连接,以提高系统的稳定性和性能。
以上就是关于“分页查询jsp代码”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
-- 展开阅读全文 --
暂无评论,1人围观