如何使用ArcGIS JS实现弹出窗口功能?
ArcGIS JS 弹出窗口详解
ArcGIS JavaScript API 提供了丰富的功能来创建和自定义地图应用。Popup
是一个重要的组件,用于在地图上显示信息窗口,本文将详细介绍如何使用 ArcGIS JS API 创建和管理Popup
。
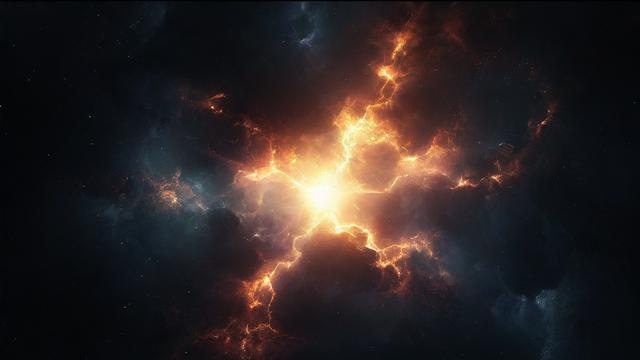
1. 什么是 Popup?
Popup
是一个信息窗口,当用户点击地图上的要素时,可以弹出显示详细信息,它支持文本、HTML、图片、视频等多种形式的内容。
2. 创建一个简单的 Popup
我们需要引入 ArcGIS JavaScript API,并创建一个基础的地图应用。
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>ArcGIS JS Popup Example</title> <link rel="stylesheet" href="https://js.arcgis.com/4.25/esri/themes/light/main.css"> <script src="https://js.arcgis.com/4.25/"></script> <style> html, body, #viewDiv { padding: 0; margin: 0; height: 100%; width: 100%; } </style> </head> <body> <div id="viewDiv"></div> <script> require([ "esri/Map", "esri/views/MapView", "esri/layers/FeatureLayer", "esri/widgets/Popup" ], function(Map, MapView, FeatureLayer, Popup) { const map = new Map({ basemap: "streets" }); const view = new MapView({ container: "viewDiv", map: map, center: [-118.80500, 34.02700], // Longitude, latitude zoom: 13 }); const layer = new FeatureLayer({ url: "https://services.arcgis.com/P3ePLMY469wqcebx.arcgis.com/arcgis/rest/services/SampleWorldCities/MapServer" }); view.when(function() { // Create a simple marker symbol and add it to the map view const symbol = { type: "simple-marker", color: "orange", size: "10px", outline: { color: "black", width: 1 } }; view.graphics.add({ geometry: { type: "point", longitude: -118.80500, latitude: 34.02700 }, symbol: symbol, popupTemplate: { title: "Sample Point", content: "This is a sample point." } }); }); }); </script> </body> </html>
3. 自定义 Popup 内容
可以通过popupTemplate
属性自定义Popup
的内容,以下是一些常用的属性:
4. 使用图层的 PopupTemplate 如果图层本身已经定义了 5. 动态更新 Popup 内容 可以在运行时动态更新 6. Popup 样式定制 可以使用 CSS 自定义 7. 常见问题与解答 问题1:如何关闭 Popup? 答:可以通过调用 问题2:如何禁用默认的点击事件触发 Popup? 答:可以通过设置图层的 通过以上步骤和示例,您应该能够熟练地在 ArcGIS JavaScript API 中创建和管理 以上内容就是解答有关“arcgis js 弹出”的详细内容了,我相信这篇文章可以为您解决一些疑惑,有任何问题欢迎留言反馈,谢谢阅读。title
content
(可以是 HTML 字符串)mediaInfos
:媒体信息数组,如图片或视频actions
:操作按钮数组
const symbol = { type: "simple-marker", color: "orange", size: "10px", outline: { color: "black", width: 1 } };
view.graphics.add({
geometry: { type: "point", longitude: -118.80500, latitude: 34.02700 },
symbol: symbol,
popupTemplate: new PopupTemplate({
title: "Custom Popup",
content: "<strong>This is a custom HTML content</strong><br/>You can include <a href='https://www.example.com' target='_blank'>links</a> too!",
mediaInfos: [{
type: "iframe",
value: {
url: "https://www.youtube.com/embed/dQw4w9WgXcQ"
}
}],
actions: [{
title: "Learn More",
url: "https://www.example.com",
target: "_blank"
}]
})
});
PopupTemplate
,则可以直接使用,使用FeatureLayer
加载一个包含PopupTemplate
的服务。
const layer = new FeatureLayer({
url: "https://services.arcgis.com/P3ePLMY469wqcebx.arcgis.com/arcgis/rest/services/SampleWorldCities/FeatureServer/0",
popupTemplate: { // This will override the default popup template if provided in the service
title: "City Name",
content: "{POP_CNTR} people live here."
}
});
Popup
的内容,通过监听click
事件来更新Popup
。
view.when(function() {
view.on("click", function(event) {
view.hitTest(event).then(function(response) {
if (response.results.length) {
const graphic = response.results[0].graphic;
if (graphic) {
graphic.popupTemplate = new PopupTemplate({
title: graphic.attributes.NAME,
content:
${graphic.attributes.POP_CNTR} people live here.
});
view.popup.open({
title: graphic.attributes.NAME,
location: event.mapPoint,
content:${graphic.attributes.POP_CNTR} people live here.
});
}
} else {
view.popup.open({ location: event.mapPoint });
}
});
});
});Popup
的样式,改变背景颜色和字体样式。
/* Custom styles for Popup */
.esri-popup .esri-widget__header {
background-color: #444;
color: white;
}
.esri-popup .esri-widget__content {
background-color: white;
color: #333;
font-family: Arial, sans-serif;
}
view.popup.close()
方法关闭Popup
。
view.when(function() {
view.popup.close();
});
outFields
属性为空数组来禁用默认的点击事件触发Popup
。
const layer = new FeatureLayer({
url: "https://services.arcgis.com/P3ePLMY469wqcebx.arcgis.com/arcgis/rest/services/SampleWorldCities/FeatureServer/0",
outFields: ["*"], // Set this to an empty array to disable default click event triggering Popup
popupTemplate: { title: "City Name", content: "{POP_CNTR} people live here." }
});
Popup
,如果您有更多问题,欢迎继续提问!
暂无评论,1人围观