在JavaScript中,如何使用foreach循环遍历数组并获取索引?
foreach JS 索引
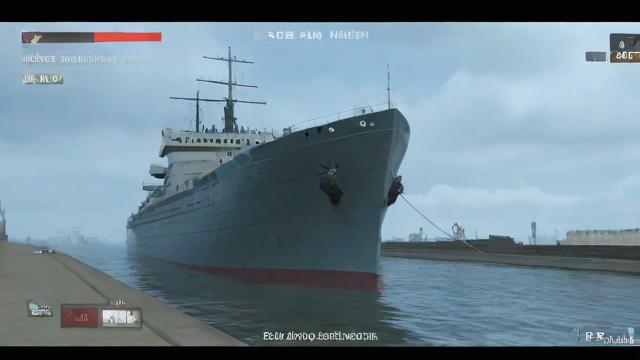
在 JavaScript 中,forEach
方法是一种遍历数组的便捷方式,它允许你对数组中的每个元素执行一个提供的函数,并且不会改变原始数组,本文将详细介绍forEach
方法的使用,包括其语法、参数、示例以及一些常见的注意事项。
1. 基本语法
array.forEach(function(currentValue, index, array) { // 执行操作 });
currentValue: 当前正在处理的元素的值。
index(可选): 当前正在处理的元素的索引。
array(可选): 调用forEach
的数组本身。
2. 示例代码
示例 1:简单遍历数组
const numbers = [1, 2, 3, 4, 5]; numbers.forEach(function(number) { console.log(number); });
输出:
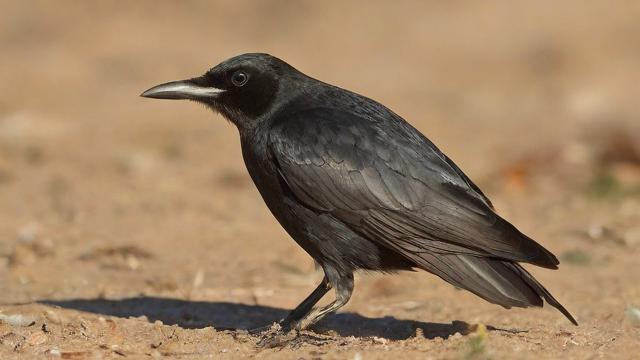
1 2 3 4 5
示例 2:使用索引
const fruits = ['apple', 'banana', 'cherry']; fruits.forEach(function(fruit, index) { console.log(index + ': ' + fruit); });
输出:
0: apple 1: banana 2: cherry
示例 3:访问整个数组
const colors = ['red', 'green', 'blue']; colors.forEach(function(color, index, array) { console.log('Color at index ' + index + ' is ' + color); console.log('Array: ' + array); });
输出:
Color at index 0 is red Array: red,green,blue Color at index 1 is green Array: red,green,blue Color at index 2 is blue Array: red,green,blue
3. 注意事项
1 不修改原数组
forEach
不会改变原数组,它只是对数组进行遍历和操作,如果你需要修改数组,可以考虑使用map
或reduce
。
2 异步操作
由于forEach
是同步执行的,如果需要在回调函数中进行异步操作,可能需要使用其他方法如for...of
或Promise
。
3 性能问题
对于大数组,forEach
的性能可能不如传统的for
循环,因为每次迭代都会创建一个新的函数作用域。
4. 相关问题与解答
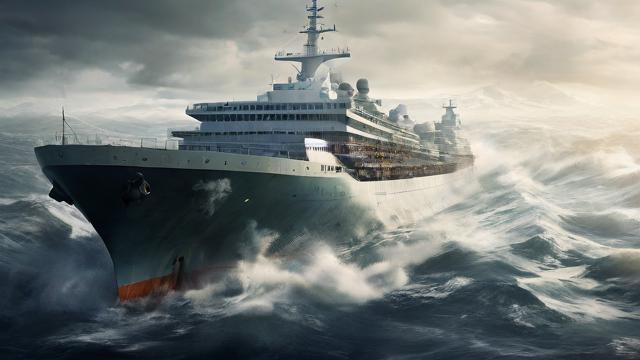
问题 1:如何在forEach
中使用this
?
在forEach
回调函数中,this
的值取决于你如何调用forEach
,默认情况下,this
指向全局对象(在浏览器中是window
),如果你想在回调函数中使用特定的this
值,可以使用Function.prototype.bind
或者箭头函数。
示例:
const obj = { value: 42, printValue() { [1, 2, 3].forEach(function(num) { console.log(this.value); // undefined (因为 this 指向全局对象) }, this); // 传递 this 作为第二个参数 } }; obj.printValue();
输出:
42 42 42
或者使用箭头函数:
const obj = { value: 42, printValue() { [1, 2, 3].forEach((num) => { console.log(this.value); // 42 (因为箭头函数继承外部的 this) }); } }; obj.printValue();
输出:
42 42 42
问题 2:forEach
是否可以中途停止?
forEach
没有内置的方法来中途停止遍历,如果你需要在某个条件下停止遍历,可以考虑使用传统的for
循环或者some
、every
等方法。
示例:使用for
循环提前退出
const numbers = [1, 2, 3, 4, 5]; for (let i = 0; i < numbers.length; i++) { if (numbers[i] === 3) break; // 当遇到3时停止遍历 console.log(numbers[i]); }
输出:
1 2
通过以上内容,我们详细介绍了forEach
方法的使用及其相关注意事项,并通过示例展示了如何正确使用forEach
,希望这些信息对你有所帮助!
各位小伙伴们,我刚刚为大家分享了有关“foreach js 索引”的知识,希望对你们有所帮助。如果您还有其他相关问题需要解决,欢迎随时提出哦!
暂无评论,1人围观