如何利用Python的存储API进行数据管理?
Python存储API
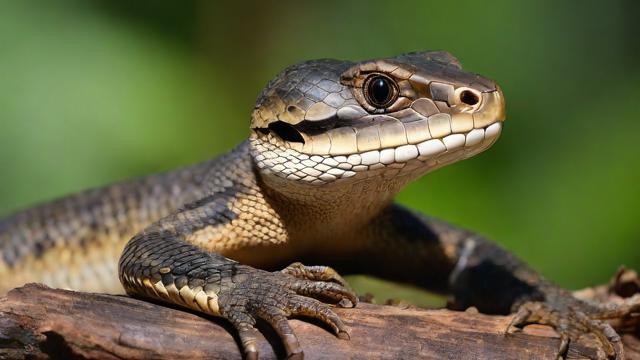
背景和简介
随着数据驱动的时代的到来,处理和存储海量数据已成为关键需求,高性能数据存储不仅能够确保数据的快速读写,还能提升系统的整体性能,Python作为一种灵活且功能强大的编程语言,提供了多种高效的数据存储解决方案,本文将详细介绍如何使用Python实现高性能数据存储,并通过具体代码示例展示其实现过程。
选择合适的存储方案
1.关系型数据库(如MySQL, PostgreSQL)
关系型数据库使用表格形式存储数据,支持复杂的查询操作,适合需要高度结构化的数据存储,MySQL和PostgreSQL都是流行的关系型数据库。
示例:使用MySQL存储数据
import mysql.connector 连接到数据库 cnx = mysql.connector.connect(user='username', password='password', host='localhost', database='test') cursor = cnx.cursor() 创建表 create_table_query = """ CREATE TABLE IF NOT EXISTS employees ( emp_id INT AUTO_INCREMENT PRIMARY KEY, first_name VARCHAR(255) NOT NULL, last_name VARCHAR(255) NOT NULL, hire_date DATE NOT NULL ) """ cursor.execute(create_table_query) 插入数据 add_employee = ("John", "Doe", '2021-09-01') insert_query = "INSERT INTO employees (first_name, last_name, hire_date) VALUES (%s, %s, %s)" cursor.execute(insert_query, add_employee) 提交事务 cnx.commit() 关闭连接 cursor.close() cnx.close()
2.非关系型数据库(如MongoDB, Redis)
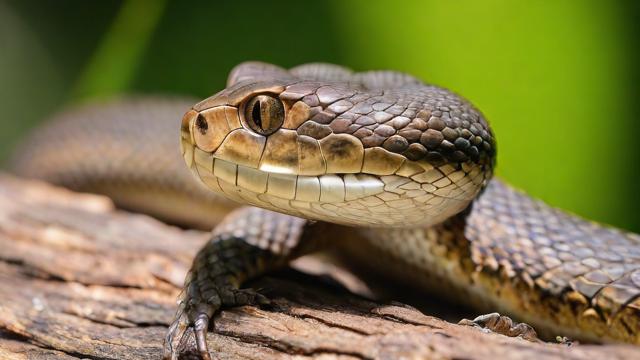
非关系型数据库适用于存储非结构化或半结构化的数据,提供高性能和高可扩展性,MongoDB是一个文档型NoSQL数据库,Redis是一个内存中的键值存储系统。
示例:使用MongoDB存储数据
from pymongo import MongoClient 连接到MongoDB client = MongoClient('localhost', 27017) db = client['test_database'] collection = db['employees'] 插入数据 employee = {"first_name": "John", "last_name": "Doe", "hire_date": "2021-09-01"} collection.insert_one(employee)
示例:使用Redis存储数据
import redis 连接到Redis r = redis.Redis(host='localhost', port=6379, db=0) 设置键值对 r.set('employee:1', 'John Doe')
3.对象存储(如Amazon S3, Google Cloud Storage)
对象存储适用于大规模数据存储,可以存储任意类型的数据,包括文件、图片、视频等,Amazon S3和Google Cloud Storage是常见的对象存储服务。
示例:使用Minio进行对象存储
from minio import Minio from minio.error import S3Error client = Minio( "play.min.io", access_key="YOURACCESSKEY", secret_key="YOURSECRETKEY", secure=True ) bucket_name = "my-bucket" object_name = "my-object" file_data = b"Hello, World!" 上传文件到MinIO try: client.put_object(bucket_name, object_name, file_data, len(file_data), part_size=10*1024*1024) print("Successfully uploaded data to MinIO") except S3Error as exc: print("Error occurred:", exc)
Python与假设的Sora AI API交互的知识点
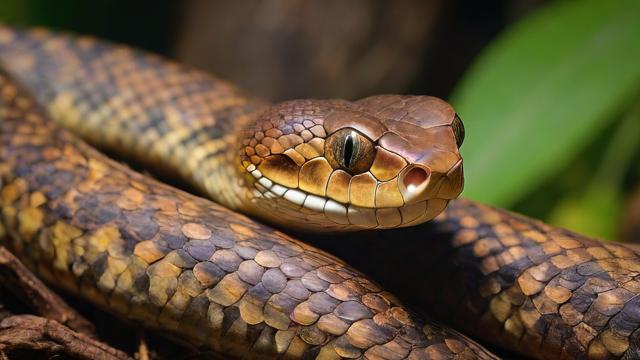
在本示例中,我们使用了Python中的requests
库来进行HTTP请求操作。requests
是一个非常流行的Python库,用于简化HTTP请求,它支持发送GET、POST、PUT、DELETE等各种HTTP请求,并且可以方便地处理响应数据。
示例:调用AI服务API的完整示例
import requests import os API_URL = "https://api.aiservice.com/v1/predict" def call_ai_service(data): headers = { 'Authorization': f'Bearer {os.getenv("AI_SERVICE_API_KEY")}', 'Content-Type': 'application/json' } try: response = requests.post(API_URL, headers=headers, json=data) if response.status_code == 200: return response.json() else: response.raise_for_status() except requests.exceptions.RequestException as e: print(f"Request failed: {e}") return None data = {"input": "你的输入数据"} result = call_ai_service(data) print(result)
归纳与进一步学习资源
在这篇文章中,我们讨论了如何使用Python调用AI服务的API,包括从选择合适的API开始,到实际调用时的注意事项,通过遵循这些步骤和建议,您可以更轻松地将AI能力集成到您的应用中,以下是一些进一步的学习资源:
[Python官方文档](https://docs.python.org/3/)
[Requests库文档](https://requests.readthedocs.io/en/latest/)
[Flask框架](https://flask.palletsprojects.com/)
[FastAPI框架](https://fastapi.tiangolo.com/)
[Django框架](https://www.djangoproject.com/)
[LeanCloud开发者文档](https://leancloud.cn/docs/)
[阿里云OSS文档](https://help.aliyun.com/document_detail/50461.html)
[腾讯云COS文档](https://cloud.tencent.com/document/product/436)
[七牛云Kodo文档](https://developer.qiniu.com/kodo/)
[又拍云USS文档](https://www.upyun.com/docs)
[AWS S3文档](https://aws.amazon.com/s3/)
[Google Cloud Storage文档](https://cloud.google.com/storage)
[Azure Blob Storage文档](https://azure.microsoft.com/en-us/services/storage/blobs/)
以上内容就是解答有关“存储apipython”的详细内容了,我相信这篇文章可以为您解决一些疑惑,有任何问题欢迎留言反馈,谢谢阅读。
暂无评论,1人围观