如何在App中实现文件上传至服务器?
以下是关于如何将文件从移动应用上传到服务器的详细步骤,假设你正在开发一个 Android 应用程序,并希望将用户选择的文件(例如图片、文档等)上传到远程服务器。
前提条件
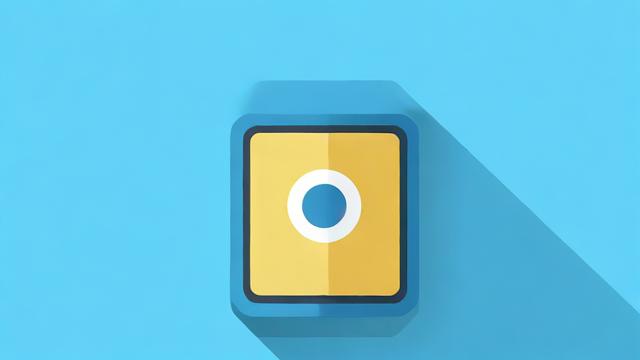
1、服务器端设置:确保你的服务器已经配置好可以接受文件上传,通常使用 HTTP POST 请求来处理文件上传。
2、网络权限:在AndroidManifest.xml
中添加必要的网络权限。
<uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" /> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
3、依赖库:如果你使用的是 Retrofit 和 OkHttp,请确保在你的build.gradle
文件中添加了相关依赖。
implementation 'com.squareup.retrofit2:retrofit:2.9.0' implementation 'com.squareup.retrofit2:converter-gson:2.9.0' implementation 'com.squareup.okhttp3:logging-interceptor:4.9.0'
步骤 1:创建文件选择器
创建一个方法让用户可以从设备中选择一个文件。
private void openFileChooser() { Intent intent = new Intent(Intent.ACTION_GET_CONTENT); intent.setType("*/*"); intent.addCategory(Intent.CATEGORY_OPENABLE); try { startActivityForResult(Intent.createChooser(intent, "Select a File to Upload"), FILE_SELECT_CODE); } catch (android.content.ActivityNotFoundException ex) { // Potentially handle the error here } }
步骤 2:处理文件选择结果
在onActivityResult
方法中处理用户选择的文件。
@Override protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) { super.onActivityResult(requestCode, resultCode, data); if (requestCode == FILE_SELECT_CODE && resultCode == RESULT_OK && data != null && data.getData() != null) { Uri fileUri = data.getData(); uploadFile(fileUri); } }
步骤 3:上传文件到服务器
使用 Retrofit 或 OkHttp 上传文件,以下是使用 Retrofit 的示例。
定义 API 接口
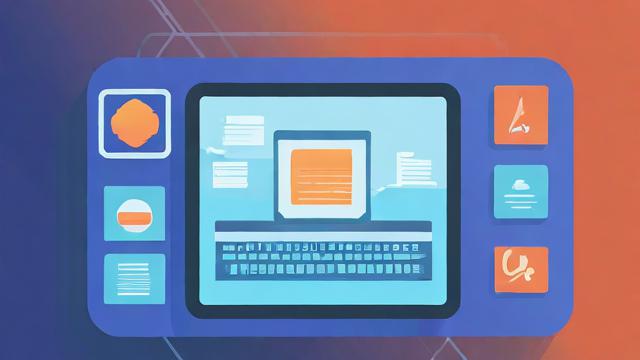
public interface ApiService { @Multipart @POST("upload") Call<ResponseBody> uploadFile(@Part MultipartBody.Part file); }
创建 Retrofit 实例
Retrofit retrofit = new Retrofit.Builder() .baseUrl("https://yourserver.com/") .addConverterFactory(GsonConverterFactory.create()) .build(); ApiService apiService = retrofit.create(ApiService.class);
准备文件部分并调用 API
private void uploadFile(Uri fileUri) { File file = new File(fileUri.getPath()); RequestBody requestFile = RequestBody.create(MediaType.parse("image/*"), file); MultipartBody.Part body = MultipartBody.Part.createFormData("file", file.getName(), requestFile); Call<ResponseBody> call = apiService.uploadFile(body); call.enqueue(new Callback<ResponseBody>() { @Override public void onResponse(Call<ResponseBody> call, Response<ResponseBody> response) { if (response.isSuccessful()) { Log.d("Upload", "File uploaded successfully!"); } else { Log.e("Upload", "File upload failed!"); } } @Override public void onFailure(Call<ResponseBody> call, Throwable t) { Log.e("Upload", "Error: " + t.getMessage()); } }); }
是一个简单的示例,展示了如何在 Android 应用中选择文件并将其上传到服务器,实际应用中可能需要更多的错误处理和优化,例如处理大文件上传、进度显示、网络变化等情况,希望这个示例对你有所帮助!
以上就是关于“app上传文件到服务器”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
-- 展开阅读全文 --
暂无评论,1人围观