Bootstrap如何处理数据库操作?
Bootstrap 处理数据库
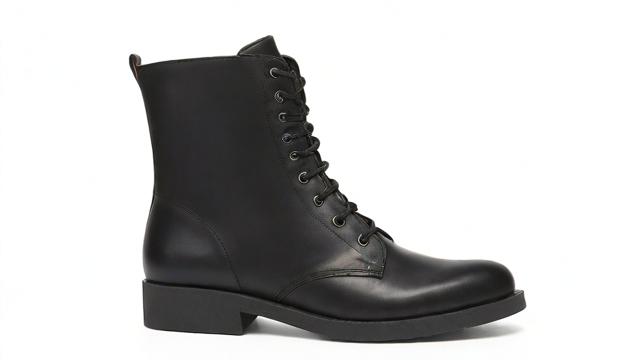
在现代Web开发中,前端框架如Bootstrap与后端数据库的结合使用是常见的做法,Bootstrap主要负责前端的样式和布局,而后端数据库则负责数据的存储和管理,本文将详细介绍如何使用Bootstrap与后端数据库进行交互,包括数据获取、展示、更新和删除等操作。
1. Bootstrap简介
Bootstrap是一个开源的前端框架,由Twitter公司推出,用于快速开发Web应用程序,它提供了丰富的CSS和JavaScript组件,帮助开发者构建响应式、美观且功能丰富的网页。
1 Bootstrap的优势
响应式设计:支持各种设备和屏幕尺寸。
丰富的组件:提供按钮、表单、导航栏等多种常用组件。
易于定制:通过自定义样式和主题,可以满足不同的设计需求。
2. 后端数据库简介
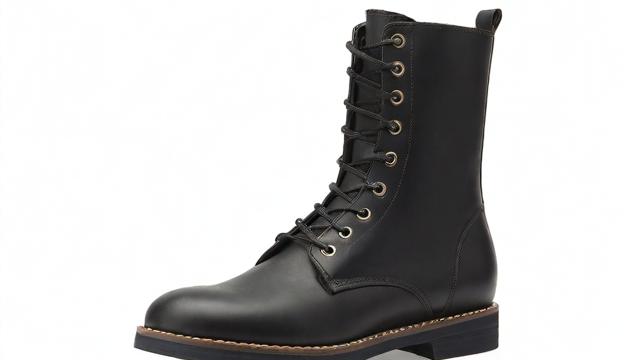
后端数据库用于存储和管理应用程序的数据,常见的后端数据库有MySQL、PostgreSQL、MongoDB等,本文将以MySQL为例进行介绍。
1 MySQL简介
MySQL是一种关系型数据库管理系统(RDBMS),广泛用于Web应用的数据存储,它具有以下特点:
开源免费:MySQL是开源软件,可以免费使用。
高性能:适用于大规模数据处理。
跨平台支持:可以在Windows、Linux、Unix等多种操作系统上运行。
3. 前后端交互方式
前后端交互通常通过API接口实现,前端发送HTTP请求到后端服务器,后端处理请求并与数据库交互,最后返回数据给前端。
1 API接口设计
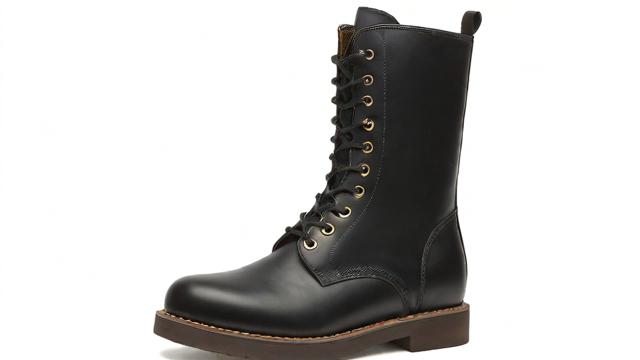
API接口是前后端通信的桥梁,设计良好的API接口可以提高系统的可维护性和扩展性,常见的RESTful API设计风格如下:
GET:获取资源
POST:创建资源
PUT:更新资源
DELETE:删除资源
2 示例:获取用户列表
假设我们需要从数据库中获取用户列表,可以通过以下步骤实现:
1、前端发送GET请求:
fetch('/api/users') .then(response => response.json()) .then(data => { console.log(data); });
2、后端处理请求:
from flask import Flask, jsonify import pymysql app = Flask(__name__) @app.route('/api/users', methods=['GET']) def get_users(): conn = pymysql.connect(host='localhost', user='root', password='password', db='test_db') cursor = conn.cursor() cursor.execute('SELECT * FROM users') users = cursor.fetchall() cursor.close() conn.close() return jsonify(users) if __name__ == '__main__': app.run(debug=True)
4. 数据展示与交互
获取到数据后,可以使用Bootstrap组件进行展示和交互,展示用户列表并实现分页功能。
1 用户列表展示
使用Bootstrap的表格组件展示用户列表:
<table class="table table-striped"> <thead> <tr> <th>User ID</th> <th>User Name</th> <th>Email</th> </tr> </thead> <tbody id="userTableBody"> <!-动态插入数据 --> </tbody> </table>
2 分页功能实现
使用JavaScript实现分页功能:
const itemsPerPage = 10;
let currentPage = 1;
const totalItems = data.length;
const totalPages = Math.ceil(totalItems / itemsPerPage);
function renderTable(page) {
const start = (page 1) * itemsPerPage;
const end = start + itemsPerPage;
const itemsToRender = data.slice(start, end);
const tableBody = document.getElementById('userTableBody');
tableBody.innerHTML = ''; // 清空表格内容
itemsToRender.forEach(item => {
const row = document.createElement('tr');
row.innerHTML =<td>${item.id}</td><td>${item.name}</td><td>${item.email}</td>
;
tableBody.appendChild(row);
});
}
// 初始化表格渲染
renderTable(currentPage);
5. 数据更新与删除
除了展示数据外,还需要实现数据的更新和删除功能,这通常通过AJAX请求实现。
1 更新用户信息
前端发送PUT请求更新用户信息:
function updateUser(userId, newData) {
fetch(/api/users/${userId}
, {
method: 'PUT',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(newData)
})
.then(response => response.json())
.then(data => {
console.log('Update successful:', data);
});
}
后端处理PUT请求:
@app.route('/api/users/<int:user_id>', methods=['PUT']) def update_user(user_id): new_data = request.get_json() conn = pymysql.connect(host='localhost', user='root', password='password', db='test_db') cursor = conn.cursor() cursor.execute('UPDATE users SET name=%s, email=%s WHERE id=%s', (new_data['name'], new_data['email'], user_id)) conn.commit() cursor.close() conn.close() return jsonify({'message': 'User updated successfully'})
2 删除用户信息
前端发送DELETE请求删除用户信息:
function deleteUser(userId) {
fetch(/api/users/${userId}
, {
method: 'DELETE'
})
.then(response => response.json())
.then(data => {
console.log('Delete successful:', data);
});
}
后端处理DELETE请求:
@app.route('/api/users/<int:user_id>', methods=['DELETE']) def delete_user(user_id): conn = pymysql.connect(host='localhost', user='root', password='password', db='test_db') cursor = conn.cursor() cursor.execute('DELETE FROM users WHERE id=%s', (user_id,)) conn.commit() cursor.close() conn.close() return jsonify({'message': 'User deleted successfully'})
相关问题与解答
问题1:如何在Bootstrap中使用模态框进行数据编辑?
解答:可以使用Bootstrap的模态框组件来实现数据编辑功能,以下是一个简单的示例:
1、HTML部分:定义模态框结构。
<div class="modal fade" id="editModal" tabindex="-1" role="dialog" aria-labelledby="editModalLabel" aria-hidden="true"> <div class="modal-dialog" role="document"> <div class="modal-content"> <div class="modal-header"> <h5 class="modal-title" id="editModalLabel">Edit User</h5> <button type="button" class="close" data-dismiss="modal" aria-label="Close"> <span aria-hidden="true">×</span> </button> </div> <div class="modal-body"> <form id="editForm"> <div class="form-group"> <label for="editName">Name</label> <input type="text" class="form-control" id="editName" placeholder="Enter name"> </div> <div class="form-group"> <label for="editEmail">Email</label> <input type="email" class="form-control" id="editEmail" placeholder="Enter email"> </div> </form> </div> <div class="modal-footer"> <button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button> <button type="button" class="btn btn-primary" id="saveChanges">Save changes</button> </div> </div> </div> </div>
2、JavaScript部分:实现模态框的显示和数据提交。
document.getElementById('saveChanges').addEventListener('click', function() { const name = document.getElementById('editName').value; const email = document.getElementById('editEmail').value; const userId = selectedUserId; // selectedUserId是从其他地方获取的用户ID updateUser(userId, { name: name, email: email }); document.getElementById('editModal').modal('hide'); });
问题2:如何提高前后端交互的安全性?
解答:提高前后端交互的安全性可以从以下几个方面入手:
1、使用HTTPS:确保所有数据传输都通过HTTPS协议进行加密,防止中间人攻击。
2、身份验证与授权:使用JWT(JSON Web Token)或其他认证机制对用户进行身份验证和授权,确保只有合法用户可以访问API。
3、输入验证:对所有用户输入进行严格验证,防止SQL注入和其他安全漏洞,使用参数化查询代替字符串拼接。
4、错误处理:合理处理错误信息,避免泄露敏感信息,不要直接返回数据库错误信息给用户。
小伙伴们,上文介绍了“bootstrap 处理数据库”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。
暂无评论,1人围观