如何通过App请求服务器获取JSON数据?
准备工作
确保你有开发环境,这通常包括以下内容:
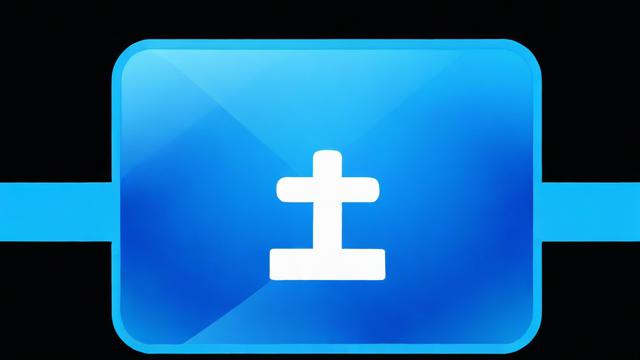
一个IDE(Android Studio、Xcode等)
一个模拟器或真实设备用于测试
网络权限配置(如果需要)
创建HTTP请求
在移动应用中,通常会使用HTTP客户端库来发送请求,对于Android,可以使用HttpURLConnection
或者第三方库如OkHttp
,对于iOS,可以使用NSURLSession
。
Android示例(使用HttpURLConnection
)
import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); new Thread(new Runnable() { @Override public void run() { try { URL url = new URL("https://api.example.com/data"); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); connection.setRequestMethod("GET"); connection.setRequestProperty("Accept", "application/json"); if (connection.getResponseCode() != 200) { throw new RuntimeException("Failed : HTTP error code : " + connection.getResponseCode()); } BufferedReader br = new BufferedReader(new InputStreamReader((connection.getInputStream()))); StringBuilder response = new StringBuilder(); String output; while ((output = br.readLine()) != null) { response.append(output); } br.close(); // Handle the JSON response here System.out.println(response.toString()); } catch (Exception e) { e.printStackTrace(); } } }).start(); } }
iOS示例(使用NSURLSession
)
import UIKit class ViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() let url = URL(string: "https://api.example.com/data")! let task = URLSession.shared.dataTask(with: url) { data, response, error in guard let data = data, error == nil else { print("Error: \(error?.localizedDescription ?? "Unknown error")") return } if let httpResponse = response as? HTTPURLResponse, httpResponse.statusCode == 200 { let jsonResponse = try? JSONSerialization.jsonObject(with: data, options: []) // Handle the JSON response here print(jsonResponse) } else { print("HTTP request failed with status code: \(response?.statusCode ?? -1)") } } task.resume() } }
处理JSON数据
解析和处理服务器返回的JSON数据,根据具体需求,你可以使用不同的JSON库,Java中常用的库有org.json
和Gson
,Swift中可以使用Codable
协议。
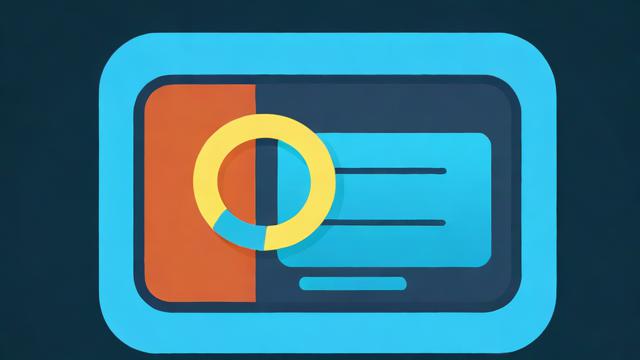
Android示例(使用org.json
)
import org.json.JSONArray;
import org.json.JSONObject;
// AssumingresponseString
contains the JSON data from server
String responseString = "{\"name\":\"John\", \"age\":30}";
try {
JSONObject jsonResponse = new JSONObject(responseString);
String name = jsonResponse.getString("name");
int age = jsonResponse.getInt("age");
// Use the extracted data
Log.d("JSON Response", "Name: " + name + ", Age: " + age);
} catch (JSONException e) {
e.printStackTrace();
}
iOS示例(使用Codable
协议)
struct User: Codable {
let name: String
let age: Int
}
// Assumingdata
is the Data object received from the server
do {
let user = try JSONDecoder().decode(User.self, from: data)
// Use the extracted data
print("Name: \(user.name), Age: \(user.age)")
} catch {
print("Error decoding JSON: \(error)")
}
错误处理和优化
错误处理:确保处理可能的网络错误和JSON解析错误。
异步操作:在主线程之外执行网络请求,以避免阻塞UI。
缓存:考虑实现缓存机制以减少网络请求次数。
安全性:确保敏感数据的安全传输,使用HTTPS。
测试和调试
在模拟器和真实设备上进行充分测试,以确保应用在不同网络条件下都能正常工作,使用工具如Charles或Postman来检查请求和响应。
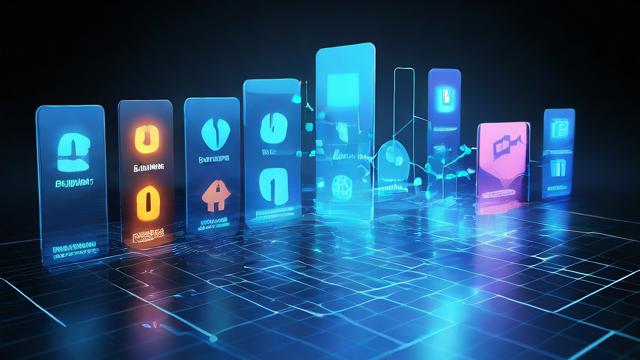
通过以上步骤,你应该能够成功从服务器请求并处理JSON数据,如果你有任何特定问题或需要进一步的帮助,请随时提问!
以上就是关于“app 请求服务器json数据”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
-- 展开阅读全文 --
暂无评论,3人围观