如何通过API获取客户端的鼠标坐标?
要通过API获取客户区鼠标坐标,你可以使用多种编程语言和框架,以下是一些常见的方法和示例代码:
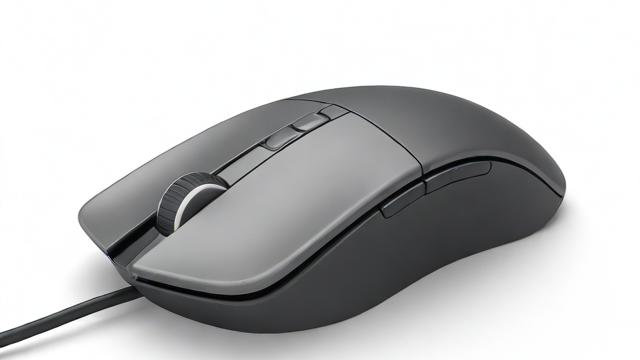
使用JavaScript在浏览器中获取鼠标坐标
如果你是在开发一个Web应用,可以使用JavaScript来获取鼠标在网页上的坐标。
document.addEventListener('mousemove', function(event) {
let x = event.clientX;
let y = event.clientY;
console.log(Mouse X: ${x}, Mouse Y: ${y}
);
});
使用Python的PyQt5库获取鼠标坐标
如果你是在使用Python进行桌面应用开发,PyQt5是一个很好的选择,以下是一个示例代码:
import sys from PyQt5.QtWidgets import QApplication, QLabel, QMainWindow from PyQt5.QtCore import Qt class MainWindow(QMainWindow): def __init__(self): super().__init__() self.label = QLabel("Move the mouse", self) self.label.setAlignment(Qt.AlignCenter) self.setCentralWidget(self.label) self.setGeometry(100, 100, 800, 600) self.setWindowTitle('Mouse Coordinates') self.mouse_moved = False def mouseMoveEvent(self, event): self.mouse_moved = True x = event.x() y = event.y() self.label.setText(f"Mouse X: {x}, Mouse Y: {y}") super().mouseMoveEvent(event) if __name__ == '__main__': app = QApplication(sys.argv) window = MainWindow() window.show() sys.exit(app.exec_())
使用Windows API(Win32 API)获取鼠标坐标
如果你在使用Windows平台,并希望通过Win32 API获取鼠标坐标,可以使用以下代码:
#include <windows.h> #include <stdio.h> int main() { POINT cursorPos; while (1) { if (GetCursorPos(&cursorPos)) { printf("Mouse X: %ld, Mouse Y: %ld ", cursorPos.x, cursorPos.y); } else { printf("Failed to get cursor position "); } Sleep(100); // 每100毫秒刷新一次坐标 } return 0; }
使用Java的Swing库获取鼠标坐标
如果你是在用Java开发桌面应用,可以使用Swing库来实现这个功能:
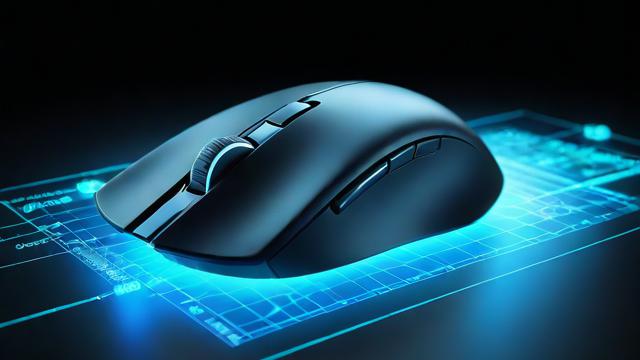
import javax.swing.*; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; public class MouseCoordinates extends JFrame { private JLabel label; public MouseCoordinates() { label = new JLabel("Move the mouse", SwingConstants.CENTER); add(label); setSize(400, 300); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setLocationRelativeTo(null); setVisible(true); addMouseMotionListener(new MouseAdapter() { @Override public void mouseMoved(MouseEvent e) { label.setText("Mouse X: " + e.getX() + ", Mouse Y: " + e.getY()); } }); } public static void main(String[] args) { new MouseCoordinates(); } }
是几种不同编程语言和环境下获取鼠标坐标的方法,希望能满足你的需求,如果你有特定的需求或遇到问题,请提供更多细节以便进一步帮助。
各位小伙伴们,我刚刚为大家分享了有关“api获得客户区鼠标坐标”的知识,希望对你们有所帮助。如果您还有其他相关问题需要解决,欢迎随时提出哦!
-- 展开阅读全文 --
短视频时代,月入过万不是梦!快来学习这些实用技巧,揭秘短视频赚钱的真实性,一起走上人生巅峰吧~💰🎬