Bootstrap Table如何实现服务器端数据查询?
使用 Bootstrap Table 实现服务器端查询数据
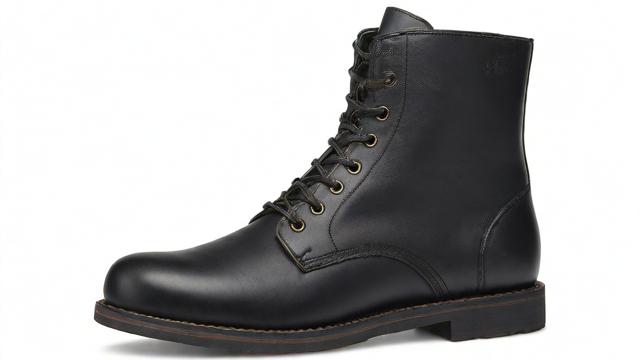
在现代 Web 开发中,前端和后端的分离已经成为一种常见的架构模式,前端负责展示数据,而后端则负责处理数据的存储和查询,这种架构不仅提高了系统的可维护性,还可以通过服务器端分页来提高性能,Bootstrap Table 是一个流行的前端表格组件,可以很方便地与服务器端进行交互,实现数据的动态加载和分页功能,本文将详细介绍如何使用 Bootstrap Table 实现服务器端查询数据。
1. 什么是 Bootstrap Table?
Bootstrap Table 是一个基于 Bootstrap 框架的表格组件,具有丰富的功能和良好的用户体验,它支持数据分页、排序、筛选、列选择等功能,并且可以轻松地与后端 API 进行交互,实现数据的动态加载和更新。
2. 安装和引入 Bootstrap Table
在使用 Bootstrap Table 之前,需要先引入相关的 CSS 和 JavaScript 文件,可以通过 CDN 或者本地文件的方式引入。
<!DOCTYPE html> <html lang="zh-CN"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Bootstrap Table 示例</title> <!-引入 Bootstrap 样式 --> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css"> <!-引入 Bootstrap Table 样式 --> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-table/1.18.3/bootstrap-table.min.css"> </head> <body> <div class="container"> <h2>Bootstrap Table 示例</h2> <table id="table" data-toggle="table" data-url="api/data"></table> </div> <!-引入 jQuery --> <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> <!-引入 Bootstrap 脚本 --> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.bundle.min.js"></script> <!-引入 Bootstrap Table 脚本 --> <script src="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-table/1.18.3/bootstrap-table.min.js"></script> </body> </html>
3. 配置 Bootstrap Table
在 HTML 中,我们通过data-toggle="table"
属性来初始化一个 Bootstrap Table。data-url
属性指定了从服务器获取数据的 API 地址。
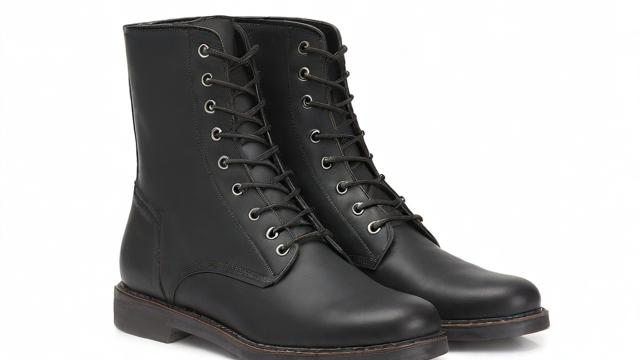
<table id="table" data-toggle="table" data-url="api/data"></table>
4. 后端 API 设计
为了实现服务器端查询数据,我们需要在后端提供一个 API,该 API 能够根据请求参数(如页码、每页条数、排序字段等)返回相应的数据,这里以一个简单的 Express.js 应用为例。
const express = require('express'); const app = express(); const port = 3000; let data = []; // 假设有一些初始数据 for (let i = 1; i <= 100; i++) { data.push({ id: i, name:Name ${i}
, age: Math.floor(Math.random() * 60) + 15 }); } app.get('/api/data', (req, res) => { const page = parseInt(req.query.page) || 1; const limit = parseInt(req.query.limit) || 10; const sort = req.query.sort ? JSON.parse(req.query.sort) : [{ field: 'id', order: 'asc' }]; const query = {}; // 根据查询条件过滤数据 if (req.query.filter) { query.name = new RegExp(req.query.filter); } // 根据排序条件排序数据 data.sort((a, b) => { for (let i = 0; i < sort.length; i++) { const field = sort[i].field; const order = sort[i].order === 'asc' ? 1 : -1; if (a[field] > b[field]) return order; if (a[field] < b[field]) return -order; } return 0; }); // 分页处理 const start = (page 1) * limit; const end = start + limit; const result = data.slice(start, end); res.json({ total: data.length, rows: result, page: page, limit: limit, sort: sort, filter: query.name ? query.name : undefined }); }); app.listen(port, () => { console.log(Server running at http://localhost:${port}/
); });
5. 前端配置分页和排序
在前端,我们可以通过配置data-side-pagination="server"
来实现服务器端分页,并通过data-sort-order
和data-sort-name
来设置默认的排序方式。
<table id="table" data-toggle="table" data-url="api/data" data-side-pagination="server" data-sort-order="asc" data-sort-name="id"></table>
6. 添加筛选功能
Bootstrap Table 还支持筛选功能,可以通过data-search="true"
属性来启用,用户输入关键词后,表格会自动发送请求到服务器进行筛选。
<input id="search" type="text" class="form-control" placeholder="搜索..."> <table id="table" data-toggle="table" data-url="api/data" data-side-pagination="server" data-sort-order="asc" data-sort-name="id"></table>
7. 完整示例
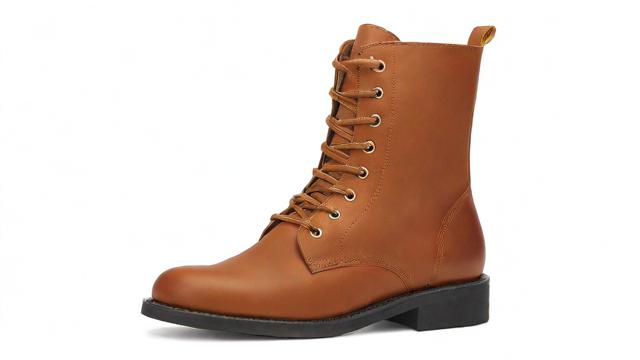
以下是一个完整的示例,包括 HTML、CSS 和 JavaScript:
<!DOCTYPE html> <html lang="zh-CN"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Bootstrap Table 示例</title> <!-引入 Bootstrap 样式 --> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css"> <!-引入 Bootstrap Table 样式 --> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-table/1.18.3/bootstrap-table.min.css"> </head> <body> <div class="container"> <h2>Bootstrap Table 示例</h2> <input id="search" type="text" class="form-control" placeholder="搜索..."> <table id="table" data-toggle="table" data-url="api/data" data-side-pagination="server" data-sort-order="asc" data-sort-name="id"></table> </div> <!-引入 jQuery --> <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> <!-引入 Bootstrap 脚本 --> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.bundle.min.js"></script> <!-引入 Bootstrap Table 脚本 --> <script src="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-table/1.18.3/bootstrap-table.min.js"></script> </body> </html>
相关问题与解答
问题1:如何在表格中显示更多列?
答:可以在表格头部定义更多的<th>
标签,并在对应的数据对象中添加相应的字段。
<thead> <tr> <th data-field="id">ID</th> <th data-field="name">姓名</th> <th data-field="age">年龄</th> <th data-field="email">邮箱</th> </tr> </thead>
同时在后端返回的数据中添加相应的字段:
{ "total": 100, "rows": [ {"id": 1, "name": "张三", "age": 25, "email": "zhangsan@example.com"}, ... ], "page": 1, "limit": 10, "sort": [{"field": "id", "order": "asc"}], "filter": null }
问题2:如何自定义表格的样式?
答:可以通过 CSS 自定义表格的样式,修改表格的边框颜色和背景色:
#table { border: 1px solid #ccc; background-color: #f9f9f9; } #table th, #table td { border: 1px solid #ccc; padding: 8px; } #table th { background-color: #f1f1f1; }
小伙伴们,上文介绍了“bootstrap table 服务器端查询数据”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。
暂无评论,1人围观